In my previous blog post comments Chris asked for some Source Code so I’ve put together a sample using the UISearchBar. The App has 2 View Controllers and 2 zibs.
- HomeScreen.cs | HomeScreen.xib
- SearchResults.cs | SearchResults.xib
You can download the entire MonoDevelop Solution here: UISearchBarSample MonoDevelop Solution
What Does It Do?
UISearchBarSample accepts a zip code, passes it off to the ZipFeeder API which returns:
- City
- County
- State
- Area Codes
- Latitude
- Longitude
- CSA Name
- CBSA Name
- Region
- Time Zone
I don’t have any affiliation with ZipFeeder, they were randomly selected from ProgrammableWeb.com where you can find tons-o-api’s.
HomeScreen contains a UISearchBar that accepts the Zip Code for user defined input, it persists the user defined value in a property and pushes a new View Controller (SearchResults) to the screen.
Once loaded, the SearchResults View Controller accesses the HomeScreen property that contains the Zip Code, makes an API call to ZipFeeder and passes the zip code. ZipFeeder returns a result as JSON.
Enter a valid zip code into the UISearchBar and click the “Search” button. (note, this is a sample there is no validation code in place).
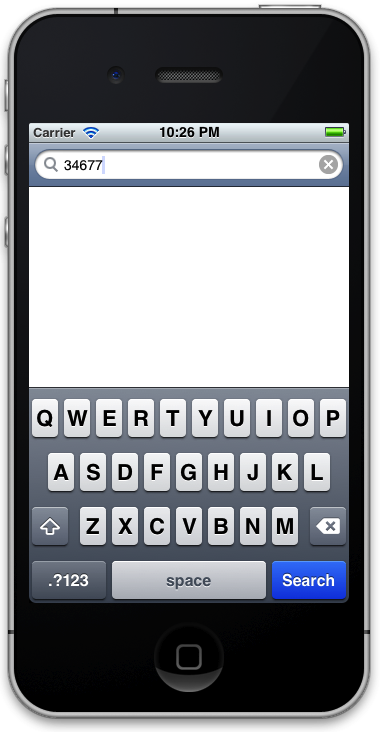
After clicking the “Search” Button, the SearchResults View Controller makes the API call to ZipFeeder and dumps the results out to the screen.
HomeScreen.cs UIViewController
Like my last example, I basically added code to ViewDidLoad() on the HomeScreen.cs ViewController to handle:
- Saving the entered search value to a property
- Dismissing the keyboard when clicking the Search Button
- Pushing the SearchResults View Controller to the screen
Saving the entered search value to a property
namespace UISearchBarSample
{
public partial class HomeScreen : UIViewController
{
//Property to hold the users search criteria;
public static string SearchCriteria;
Dismiss the Keyboard
// Dismiss the UISearcBar “Search” Button when clicked
this.uiSearchBar.SearchButtonClicked += delegate {
uiSearchBar.ResignFirstResponder ();
} ;
Push a new View Controller to the Screen
// Handle the Search Click
this.uiSearchBar.SearchButtonClicked += (sender, e) => {
// Populate SearchCriteria Property so user defined value is available on SearchResults ViewController
SearchCriteria = uiSearchBar.Text;
// Clear out user entered text
uiSearchBar.Text = string.Empty;
// Clears out previous results on SearchResults VC
searchResults = null;
// New up the SearchResults VC
if (this.searchResults == null) {
this.searchResults = new SearchResults ();
}
// display the Search Results screen
this.NavigationController.PushViewController (this.searchResults, true);
} ;
SearchResults.cs UIViewController
In the SearchResults.cs View Controller I had to:
- Add a UITextView in XCode IB
- Assign an IBOutlet to the UITextView as tvSearchResultsPanel
- Call the ZipFeeder API with the entered Zip Code
- Create HTTP Request to API
- Catch HTTP Response from API
- Dump results to SearchResults screen for user to see
These features are handled directly in the SearchResults ViewDidLoad. Note, you’ll need to get your own API key from ZipFeeder.us, the process is instant. Replace “ENTER_YOUR_KEY” with your ZipFeeder API Key
public override void ViewDidLoad ()
{
base.ViewDidLoad ();
// Inform user what they’re searching for
tvSearchResultsPanel.Text += “Searching for: \r\n” + HomeScreen.SearchCriteria + “\r\n”;
// ZIP Feeder API Call
var request = HttpWebRequest.Create (string.Format (@”http://www.zipfeeder.us/zip?key=ENTER_YOUR_KEY&zips=” + HomeScreen.SearchCriteria));
request.ContentType = “application/json”;
request.Method = “POST”;
// results text container
resultText = new StringBuilder ();
// Handle HTTP Request and HTTP Response
using (HttpWebResponse response = request.GetResponse() as HttpWebResponse) {
// dump any errors into the results container
if (response.StatusCode != HttpStatusCode.OK) {
resultText.Append (“Error fetching result. Server returned status code: ” + response.StatusCode + “\r\n”);
}
// catch the response and dump into the result text container
using (StreamReader reader = new StreamReader(response.GetResponseStream())) {
var content = reader.ReadToEnd ();
if (string.IsNullOrWhiteSpace (content)) {
resultText.Append (“Response contained an empty body.\r\n”);
} else {
resultText.Append (“Response body: \r\n” + content);
}
}
}
// populate the UITextView with the results container value
tvSearchResultsPanel.Text += resultText.ToString();
}